Getting Started#
To get things started we will try to run a very simple GTK based GUI application using the PyGObject provided
Python bindings. First create a small Python script called hello.py
with
the following content and save it somewhere:
import sys
import gi
gi.require_version("Gtk", "4.0")
from gi.repository import GLib, Gtk
class MyApplication(Gtk.Application):
def __init__(self):
super().__init__(application_id="com.example.MyGtkApplication")
GLib.set_application_name('My Gtk Application')
def do_activate(self):
window = Gtk.ApplicationWindow(application=self, title="Hello World")
window.present()
app = MyApplication()
exit_status = app.run(sys.argv)
sys.exit(exit_status)
Before we can run the example application we need to install PyGObject, GTK and their dependencies. Follow the instructions for your platform below.
For full IDE support (incl. autocomplete) you will also need typing stubs available here PyGObject-stubs.
After running the example application have a look at the Tutorials for an overview of how to create GTK apps and the GNOME Python API docs for API documentation for all supported libraries.
Windows#
Go to https://www.msys2.org/ and download the x86_64 installer
Follow the instructions on the page for setting up the basic environment
Run
C:\msys64\ucrt64.exe
- a terminal window should pop upExecute
pacman -Suy
Execute
pacman -S mingw-w64-ucrt-x86_64-gtk4 mingw-w64-ucrt-x86_64-python3 mingw-w64-ucrt-x86_64-python3-gobject
To test that GTK is working you can run
gtk4-demo
Copy the
hello.py
script you created toC:\msys64\home\<username>
In the mingw32 terminal execute
python3 hello.py
- a window should appear.

Ubuntu / Debian#
- Installing the system provided PyGObject:
Open a terminal
Execute
sudo apt install python3-gi python3-gi-cairo gir1.2-gtk-4.0
Change the directory to where your
hello.py
script can be found (e.g.cd Desktop
)Run
python3 hello.py
- Installing from PyPI with pip:
Open a terminal and enter your virtual environment
Execute
sudo apt install libgirepository-2.0-dev gcc libcairo2-dev pkg-config python3-dev gir1.2-gtk-4.0
to install the build dependencies and GTKExecute
pip3 install pycairo
to build and install PycairoExecute
pip3 install PyGObject
to build and install PyGObjectChange the working directory to where your
hello.py
script can be foundRun
python3 hello.py
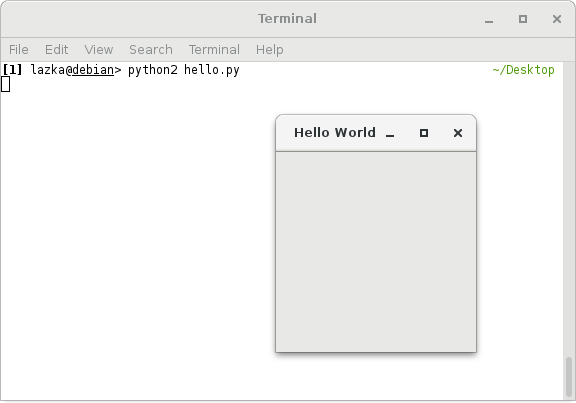
Fedora#
- Installing the system provided PyGObject:
Open a terminal
Execute
sudo dnf install python3-gobject gtk4
Change the working directory to where your
hello.py
script can be foundRun
python3 hello.py
- Installing from PyPI with pip:
Open a terminal and enter your virtual environment
Execute
sudo dnf install gcc gobject-introspection-devel cairo-gobject-devel pkg-config python3-devel gtk4
to install the build dependencies and GTKExecute
pip3 install pycairo
to build and install PycairoExecute
pip3 install PyGObject
to build and install PyGObjectChange the working directory to where your
hello.py
script can be foundRun
python3 hello.py
Arch Linux#
- Installing the system provided PyGObject:
Open a terminal
Execute
sudo pacman -S python-gobject gtk4
Change the working directory to where your
hello.py
script can be foundRun
python3 hello.py
- Installing from PyPI with pip:
Open a terminal and enter your virtual environment
Execute
sudo pacman -S python cairo pkgconf gobject-introspection gtk4
to install the build dependencies and GTKExecute
pip3 install pycairo
to build and install PycairoExecute
pip3 install PyGObject
to build and install PyGObjectChange the working directory to where your
hello.py
script can be foundRun
python3 hello.py
openSUSE#
- Installing the system provided PyGObject:
Open a terminal
Execute
sudo zypper install python3-gobject python3-gobject-Gdk typelib-1_0-Gtk-4_0 libgtk-4-1
Change the directory to where your
hello.py
script can be foundRun
python3 hello.py
- Installing from PyPI with pip:
Open a terminal and enter your virtual environment
Execute
sudo zypper install cairo-devel pkg-config python3-devel gcc gobject-introspection-devel
to install the build dependencies and GTKExecute
pip3 install pycairo
to build and install PycairoExecute
pip3 install PyGObject
to build and install PyGObjectChange the working directory to where your
hello.py
script can be foundRun
python3 hello.py
macOS#
Go to https://brew.sh/ and install homebrew
Open a terminal
Execute
brew install pygobject3 gtk4
Change the working directory to where your
hello.py
script can be foundRun
python3 hello.py
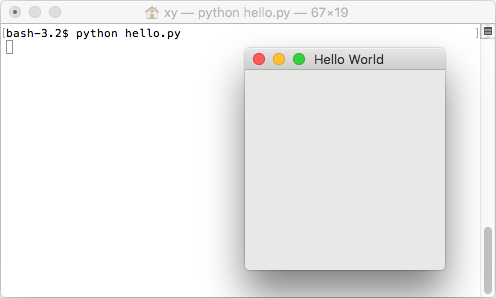
For more details on how to use a virtualenv with PyGObject, see the “Creating a Development Environment” page.