DropDown#
Gtk.DropDown
allows the user to choose an item from a list of options.
They are preferable to having many radio buttons on screen as they take up less
room.
To populate its options Gtk.DropDown
uses a Gio.ListModel
.
Gio.ListModel
it’s an interface that represents a mutable list of
GObject.Objects
. For text-only uses cases GTK provides
Gtk.StringList
, a list model that wraps an array of strings wrapped on
Gtk.StringObject
and Gtk.DropDown
knows how to use it.
Gtk.DropDown
can optionally allow search in the popup, which is useful
if the list of options is long. To enable the search entry, use
Gtk.DropDown.props.enable_search
.
Attention
If you use custom list models with custom gobjects you must provide a
Gtk.Expression
through Gtk.DropDown.props.expression
so
Gtk.DropDown
can know how to filter the gobjects.
Gtk.Expression
has been supported in PyGObject since release 3.48.
Gtk.DropDown
stores the selected item from the list model in
Gtk.DropDown.props.selected_item
, and the position of that item on
Gtk.DropDown.props.selected
. To know when the selection has changed just
connect to notify::selected-item
or notify::selected
.
Example#
We are creating a simple Gtk.DropDown
using Gtk.StringList
.
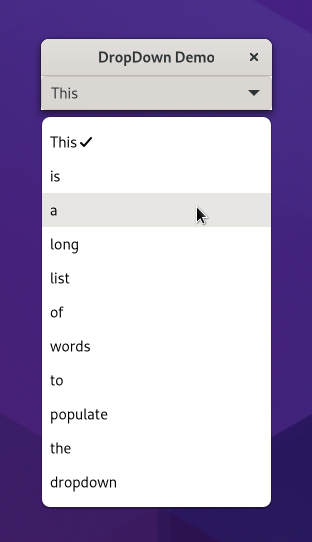
1import gi
2
3gi.require_version('Gtk', '4.0')
4from gi.repository import Gtk
5
6
7class DropDownWindow(Gtk.ApplicationWindow):
8 def __init__(self, **kargs):
9 super().__init__(**kargs, title='DropDown Demo')
10
11 dropdown = Gtk.DropDown()
12 dropdown.connect('notify::selected-item', self.on_string_selected)
13 self.set_child(dropdown)
14
15 strings = Gtk.StringList()
16 dropdown.props.model = strings
17 items = 'This is a long list of words to populate the dropdown'.split()
18
19 # Populate the list
20 for item in items:
21 strings.append(item)
22
23 def on_string_selected(self, dropdown, _pspec):
24 # Selected Gtk.StringObject
25 selected = dropdown.props.selected_item
26 if selected is not None:
27 print('Selected', selected.props.string)
28
29
30def on_activate(app):
31 win = DropDownWindow(application=app)
32 win.present()
33
34
35app = Gtk.Application(application_id='com.example.App')
36app.connect('activate', on_activate)
37
38app.run(None)