Entries#
Entries allows the user to enter text.
GTK provides a few entry widgets for different use cases.
Those are the generic Gtk.Entry
, Gtk.PasswordEntry
and
Gtk.SearchEntry
.
Although none of these widgets derive from each other, they implement the
Gtk.Editable
interface, so they share some
properties and methods.
You can change and get the contents with the Gtk.Editable.props.text
property.
You can also limit the number of characters the entry can take with
Gtk.Editable.props.max_width_chars
.
Occasionally you might want to make an Entry widget read-only. This can be done
by setting Gtk.Editable.props.editable
to False
.
Generic Entry#
Gtk.Entry
is the standard entry.
Gtk.Entry
can be set to show a invisible character using
Gtk.Entry.props.visibility
and Gtk.Entry.props.invisible_char
,
this is useful to hide the text of the entry when it is used for example to
retrieve passwords.
Though Password Entry exist for this specific use
case.
Gtk.Entry
has the ability to display progress or activity information
behind the text. This is similar to Gtk.ProgressBar
widget and is
commonly found in web browsers to indicate how much of a page download has been
completed. To make an entry display such information, use
Gtk.Entry.props.progress_fraction
,
Gtk.Entry.props.progress_pulse_step
, or Gtk.Entry.progress_pulse()
.
Additionally, a Gtk.Entry
can show icons at either side of the entry.
These icons can be activatable by clicking, can be set up as drag source and can
have tooltips.
To add an icon, use Gtk.Entry.set_icon_from_icon_name()
or one of the
various other properties and methods that set an icon from an icon name,
Gio.Icon
, or a Gdk.Paintable
.
To set a tooltip on an icon, use Gtk.Entry.set_icon_tooltip_text()
or the
corresponding function for markup.
Entries can have a placeholder text,
just use Gtk.Entry.props.placeholder_text
.
Password Entry#
Gtk.PasswordEntry
brings some features for password handing.
It can show a button to toggle the visibility of the text if you set
Gtk.PasswordEntry.props.show_peek_icon
to True
.
Search Entry#
Gtk.SearchEntry
is an entry specially designed for use as a search
entry.
Gtk.SearchEntry
allows you to implement the popular “type to search”
feature.
You can use Gtk.SearchEntry.set_key_capture_widget()
to set the parent
widget that will redirect its key events to the entry.
Gtk.SearchEntry
has the
search-changed
signal, it
differs from Gtk.Editable
’s changed
in the sense that it gets emitted after a short delay that you can configure
with Gtk.SearchEntry.props.search_delay
.
This way you can get reactive searching without over loading your backend.
Example#
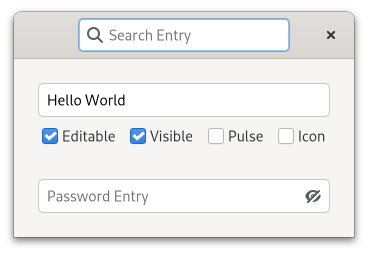
1import gi
2
3gi.require_version('Gtk', '4.0')
4from gi.repository import Gtk, GLib
5
6
7class EntryWindow(Gtk.ApplicationWindow):
8 def __init__(self, **kargs):
9 super().__init__(**kargs, title='Entry Demo')
10
11 self.set_size_request(200, 100)
12
13 self.timeout_id = None
14
15 header = Gtk.HeaderBar()
16 self.set_titlebar(header)
17
18 vbox = Gtk.Box(orientation=Gtk.Orientation.VERTICAL, spacing=6)
19 vbox.props.margin_start = 24
20 vbox.props.margin_end = 24
21 vbox.props.margin_top = 24
22 vbox.props.margin_bottom = 24
23 self.set_child(vbox)
24
25 # Gtk.SearchEntry
26 search = Gtk.SearchEntry()
27 search.props.placeholder_text = 'Search Entry'
28 search.set_key_capture_widget(self)
29 header.set_title_widget(search)
30 self.set_focus(search)
31
32 # Gtk.Entry
33 self.entry = Gtk.Entry()
34 self.entry.set_text('Hello World')
35 vbox.append(self.entry)
36
37 hbox = Gtk.Box(spacing=6)
38 vbox.append(hbox)
39
40 self.check_editable = Gtk.CheckButton(label='Editable')
41 self.check_editable.connect('toggled', self.on_editable_toggled)
42 self.check_editable.props.active = True
43 hbox.append(self.check_editable)
44
45 self.check_visible = Gtk.CheckButton(label='Visible')
46 self.check_visible.connect('toggled', self.on_visible_toggled)
47 self.check_visible.props.active = True
48 hbox.append(self.check_visible)
49
50 self.pulse = Gtk.CheckButton(label='Pulse')
51 self.pulse.connect('toggled', self.on_pulse_toggled)
52 hbox.append(self.pulse)
53
54 self.icon = Gtk.CheckButton(label='Icon')
55 self.icon.connect('toggled', self.on_icon_toggled)
56 hbox.append(self.icon)
57
58 # Gtk.PasswordEntry
59 pass_entry = Gtk.PasswordEntry()
60 pass_entry.props.placeholder_text = 'Password Entry'
61 pass_entry.props.show_peek_icon = True
62 pass_entry.props.margin_top = 24
63 vbox.append(pass_entry)
64
65 def on_editable_toggled(self, button):
66 value = button.get_active()
67 self.entry.set_editable(value)
68
69 def on_visible_toggled(self, button):
70 self.entry.props.visibility = button.props.active
71
72 def on_pulse_toggled(self, button):
73 if button.get_active():
74 self.entry.props.progress_pulse_step = 0.2
75 # Call self.do_pulse every 100 ms
76 self.timeout_id = GLib.timeout_add(100, self.do_pulse)
77 else:
78 # Don't call self.do_pulse anymore
79 GLib.source_remove(self.timeout_id)
80 self.timeout_id = None
81 self.entry.props.progress_pulse_step = 0
82
83 def do_pulse(self):
84 self.entry.progress_pulse()
85 return True
86
87 def on_icon_toggled(self, button):
88 if button.props.active:
89 icon_name = 'system-search-symbolic'
90 else:
91 icon_name = None
92 self.entry.set_icon_from_icon_name(
93 Gtk.EntryIconPosition.PRIMARY, icon_name
94 )
95
96
97def on_activate(app):
98 win = EntryWindow(application=app)
99 win.present()
100
101
102app = Gtk.Application(application_id='com.example.App')
103app.connect('activate', on_activate)
104
105app.run(None)