Popovers#
The Gtk.Popover
is an special kind of window used for displaying
additional information and is often used with button menus and context menus.
Popovers are attached to a parent widget and point to it.
It’s position can be configured with Gtk.Popover.props.position
.
Gtk.Popover
shows an arrow to visually connect where it points to,
this can be disabled setting Gtk.Popover.props.has_arrow
to False
.
A Gtk.Popover
can be opened using Gtk.Popover.popup()
and hidden
with Gtk.Widget.hide()
.
GTK also provides Gtk.MenuButton
, it’s an special button that can show
and hide a Gtk.Popover
attached to it using
Gtk.MenuButton.props.popover
.
Custom Popover#
A child widget can be added to a popover using Gtk.Popover.set_child()
.
The parent of the popover can be set using Gtk.Widget.set_parent()
.
Example#
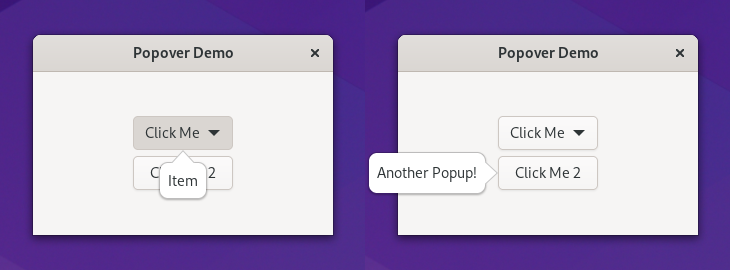
1import gi
2
3gi.require_version('Gtk', '4.0')
4from gi.repository import Gtk
5
6
7class PopoverWindow(Gtk.ApplicationWindow):
8 def __init__(self, **kargs):
9 super().__init__(**kargs, title='Popover Demo')
10
11 self.set_default_size(300, 200)
12
13 box = Gtk.Box(spacing=6, orientation=Gtk.Orientation.VERTICAL)
14 box.props.halign = box.props.valign = Gtk.Align.CENTER
15 self.set_child(box)
16
17 popover = Gtk.Popover()
18 popover_box = Gtk.Box()
19 popover_box.append(Gtk.Label(label='Item'))
20 popover.set_child(popover_box)
21
22 button = Gtk.MenuButton(label='Click Me', popover=popover)
23 box.append(button)
24
25 button2 = Gtk.Button(label='Click Me 2')
26 button2.connect('clicked', self.on_button_clicked)
27 box.append(button2)
28
29 self.popover2 = Gtk.Popover()
30 self.popover2.set_child(Gtk.Label(label='Another Popup!'))
31 self.popover2.set_parent(button2)
32 self.popover2.props.position = Gtk.PositionType.LEFT
33
34 def on_button_clicked(self, _button):
35 self.popover2.popup()
36
37
38def on_activate(app):
39 win = PopoverWindow(application=app)
40 win.present()
41
42
43app = Gtk.Application(application_id='com.example.App')
44app.connect('activate', on_activate)
45
46app.run(None)