Label#
Labels are the main method of placing non-editable text in windows, for instance
to place a title next to a Gtk.Entry
widget. You can specify the text
in the constructor, or later with the Gtk.Label.props.label
property.
The width of the label will be adjusted automatically. You can produce
multi-line labels by putting line breaks (\n
) in the label string.
Labels can be made selectable with Gtk.Label.props.selectable
.
Selectable labels allow the user to copy the label contents to the
clipboard. Only labels that contain useful-to-copy information —
such as error messages — should be made selectable.
The label text can be justified using the Gtk.Label.props.justify
prop.
The widget is also capable of word-wrapping, which can be activated with
Gtk.Label.props.wrap
. You can configure this wrapping by changing
Gtk.Label.props.wrap_mode
, in can be Pango.WrapMode.WORD
,
Pango.WrapMode.CHAR
or Pango.WrapMode.WORD_CHAR
.
Gtk.Label
support some simple formatting, for instance allowing you to
make some text bold, colored, or larger. You can do this by enabling markup
setting Gtk.Label.props.use_markup
to True
, then you can use markup
with Gtk.Label.props.label
using the Pango Markup syntax [1].
For instance, <b>bold text</b> and <s>strikethrough text</s>
.
In addition, Gtk.Label
supports clickable hyperlinks.
The markup for links is borrowed from HTML, using the a with href and title
attributes. GTK renders links similar to the way they appear in web browsers,
with colored, underlined text. The title attribute is displayed as a tooltip
on the link. Gtk.Label.set_markup()
method can be used to set the text and
enable markup at the same time.
label.set_markup("Go to <a href=\"https://www.gtk.org\" "
"title=\"Our website\">GTK+ website</a> for more")
Labels may contain mnemonics. Mnemonics are underlined characters in the
label, used for keyboard navigation. Mnemonics are created by providing a
string with an underscore before the mnemonic character, such as “_File”,
to the functions Gtk.Label.new_with_mnemonic()
or
Gtk.Label.set_text_with_mnemonic()
.
Mnemonics automatically activate any activatable widget the label is inside,
such as a Gtk.Button
; if the label is not inside the mnemonic’s target
widget, you have to tell the label about the target using
Gtk.Label.props.mnemonic_widget
.
You can also change the alignment of the label text inside its size allocation.
For example if you use Gtk.Widget.props.hexpand
in a label, the label
may take more space than its inner text.
You can change this alignment using Gtk.Label.props.xalign
for
horizontal alignment and Gtk.Label.props.yalign
for vertical.
Example#
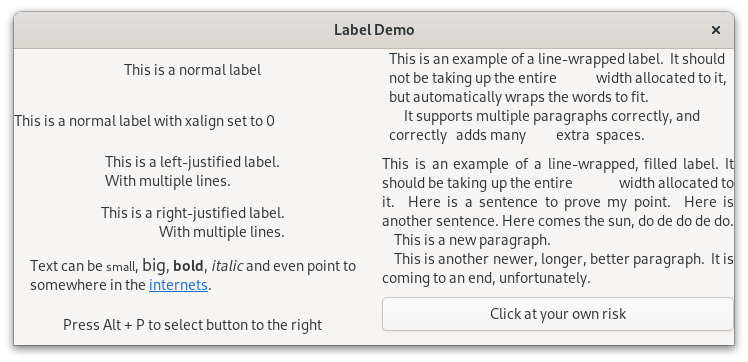
1import gi
2
3gi.require_version('Gtk', '4.0')
4from gi.repository import Gtk
5
6
7class LabelWindow(Gtk.ApplicationWindow):
8 def __init__(self, **kargs):
9 super().__init__(**kargs, title='Label Demo')
10
11 box = Gtk.Box(spacing=10)
12 self.set_child(box)
13
14 box_left = Gtk.Box(orientation=Gtk.Orientation.VERTICAL, spacing=10)
15 box_left.props.hexpand = True
16 box_left.props.homogeneous = True
17 box_right = Gtk.Box(orientation=Gtk.Orientation.VERTICAL, spacing=10)
18
19 box.append(box_left)
20 box.append(box_right)
21
22 label = Gtk.Label(label='This is a normal label')
23 box_left.append(label)
24
25 label = Gtk.Label(label='This is a normal label with xalign set to 0')
26 label.props.xalign = 0
27 box_left.append(label)
28
29 label = Gtk.Label()
30 label.props.label = (
31 'This is a left-justified label.\nWith multiple lines.'
32 )
33 label.props.justify = Gtk.Justification.LEFT
34 box_left.append(label)
35
36 label = Gtk.Label(
37 label='This is a right-justified label.\nWith multiple lines.'
38 )
39 label.props.justify = Gtk.Justification.RIGHT
40 box_left.append(label)
41
42 label = Gtk.Label(
43 label='This is an example of a line-wrapped label. It '
44 'should not be taking up the entire '
45 'width allocated to it, but automatically '
46 'wraps the words to fit.\n'
47 ' It supports multiple paragraphs correctly, '
48 'and correctly adds '
49 'many extra spaces. '
50 )
51 label.props.wrap = True
52 label.props.max_width_chars = 32
53 box_right.append(label)
54
55 label = Gtk.Label(
56 label='This is an example of a line-wrapped, filled label. '
57 'It should be taking '
58 'up the entire width allocated to it. '
59 'Here is a sentence to prove '
60 'my point. Here is another sentence. '
61 'Here comes the sun, do de do de do.\n'
62 ' This is a new paragraph.\n'
63 ' This is another newer, longer, better '
64 'paragraph. It is coming to an end, '
65 'unfortunately.'
66 )
67 label.props.wrap = True
68 label.props.justify = Gtk.Justification.FILL
69 label.props.max_width_chars = 32
70 box_right.append(label)
71
72 label = Gtk.Label()
73 label.set_markup(
74 'Text can be <small>small</small>, <big>big</big>, '
75 '<b>bold</b>, <i>italic</i> and even point to '
76 'somewhere in the <a href="https://www.gtk.org" '
77 'title="Click to find out more">internets</a>.'
78 )
79 label.props.wrap = True
80 label.props.max_width_chars = 48
81 box_left.append(label)
82
83 label = Gtk.Label.new_with_mnemonic(
84 '_Press Alt + P to select button to the right'
85 )
86 box_left.append(label)
87 label.props.selectable = True
88
89 button = Gtk.Button(label='Click at your own risk')
90 label.props.mnemonic_widget = button
91 box_right.append(button)
92
93
94def on_activate(app):
95 win = LabelWindow(application=app)
96 win.present()
97
98
99app = Gtk.Application(application_id='com.example.App')
100app.connect('activate', on_activate)
101
102app.run(None)